Factory Method Design Pattern in Java
This tutorial explains Gang of Four's Factory Method design pattern in java. It starts with the definition of the pattern, which is followed by the class diagram showing the constituent classes of the pattern, and then explains the role played by each these classes. Next an example use case of factory pattern is implemented in Java. Begining with the class diagram for the use case, Java code for its implementation is shown next, which is followed by a detailed explanation of the code.
Introduction
Factory Method Design Pattern is a creational design pattern among the Gang Of Four(GOF) Design PatternsArticle on GOF Patterns & their types. Being a creational design pattern, the factory method design pattern deals with the creation of a family of objects.
What is Factory Method Design Pattern
Factory method design pattern creates objects in such a way that it lets the sub-classes decide how to implement the object creation logic.
In the factory pattern, there is a base factory interface/base class which defines a common method for creating objects of subclasses. The actual logic for creation of different type of objects is present in the implementations/subclasses, which determine how to implement the creation logic by overriding the pre-defined instance creation method. Lets have a look at the class diagram of a factory method design pattern to understand it better.
UML Class Diagram for Factory Method Design Pattern
Explanation of the code
Explanation of Code & Class Diagram
The Java class diagram above depicts factory pattern implemented for a Document Management System. An important point to note here is that, in practice, in almost all java libraries, the
Lets understand the Java Class Diagram for Factory Method Design Pattern in detail to understand how it works -
Summary
In the above tutorial we looked at what is factory method design pattern moving on to class diagram for a factory method pattern & its explanation. We then looked at class diagram and code for a Java example of factory method pattern followed by its explanation. This concludes the tutorial on factory method design pattern in java.
In the factory pattern, there is a base factory interface/base class which defines a common method for creating objects of subclasses. The actual logic for creation of different type of objects is present in the implementations/subclasses, which determine how to implement the creation logic by overriding the pre-defined instance creation method. Lets have a look at the class diagram of a factory method design pattern to understand it better.
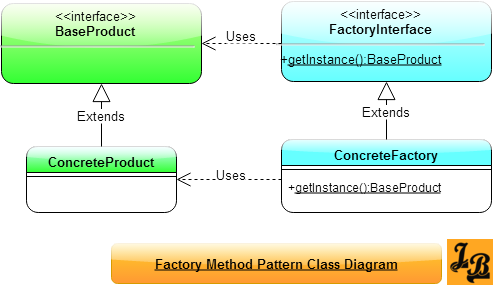
BaseProduct
is the base interfaces for all products.ConcreteProduct
implements BaseProduct. There are usually multipleConcreteProduct
implementations for aBaseProduct
when a factory design pattern is chosen for object creation.FactoryInterface
defines the basic behavior of the factory which in this case is defined by the methodgetInstance()
.ConcreteFactory
implementsFactoryInterface
and provides implementation for thegetInstance()
method. There can be mutipleConcreteFactory
sub-classes.- The
ConcreteFactory
’sgetInstance()
method creates an object of one of theConcreteProduct
classes. It however sends back an object of typeBaseProduct
. - The actual object created is as per the client invocation context. I.e. if the client asks
ConcreteFactory
to giveConcreteProduct
of say type-X,getInstance()
sends back an object of that type. If the client asks for type-Y, then it gets back an object of that type and so on. - The object creation method
getInstance()
ofConcreteFactory
is thus standardized and it creates objects ofConcreteProduct
set of classes while returning the object in aBaseProduct
reference. This is the Factory Method Design Pattern.
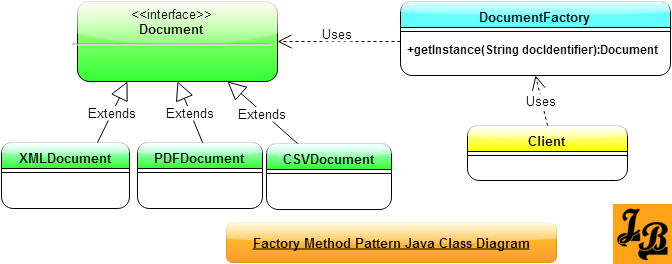
Code for the classes shown in Java Example's Class Diagram
//Document.java
public interface Document{
//Specification of methods specific to a Document
//is done here
}
//PDFDocument.java,XMLDocument.java and CSVDocument.java
public class PDFDocument implements Document{
//Code for overriding Document methods
}
public class XMLDocument implements Document{
//Code for overriding Document methods
}
public class CSVDocument implements Document{
//Code for overriding Document methods
}
//DocumentFactory.java
public class DocumentFactory{
public Document getInstance(String docIdentifier){
switch(docIdentifier){
case "XML": return new XMLDocument();
case "PDF": return new PDFDocument();
case "CSV": return new CSVDocument();
}
return null;
}
}
//Client.java
public class Client{
public static void main(String args[]){
//creates an instance of XML document
XMLDocument xmlDocument=(XMLDocument) new DocumentFactory().getInstance("XML");
//Similarly creates an instance of PDF document
PDFDocument pdfDocument=(PDFDocument) new DocumentFactory().getInstance("PDF");
}
}
FactoryInterface
(as explained in section 'What is Factory Pattern' above) is not created. I.e, The Java implementation directly uses a ConcreteFactory
which creates the ConcreteProduct
instances.Lets understand the Java Class Diagram for Factory Method Design Pattern in detail to understand how it works -
- Interface
Document
is the base product interface. XMLProduct
,CSVProduct
andPDFProduct
are concrete implementations ofDocument
interface.DocumentFactory
has agetInstance()
method which takes as input the stringdocIdentifier
. Its through thedocIdentifier
parameter that theClient
conveys the message toDocumentFactory
regarding which type of document it needs.DocumentFactory
creates the required implementation ofDocument
and returns the object back as typeDocument
. Thus, thegetInstance()
creates objects ofDocument
’s implementation classes based on client needs. This is how the Factory Method Design Pattern works.
Gang of Four (GOF) Design Patterns on JavaBrahman
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern
Creational Patterns: Factory method PatternFactory Method Design Pattern in Java Builder PatternBuilder Design Pattern in Java Prototype PatternPrototype Design Pattern in Java
Behavioral Patterns: Chain of Responsibility PatternChain of Responsibility Design Pattern in Java Observer PatternObserver Design Pattern in Java Iterator PatternIterator Design Pattern in Java State PatternState Design Pattern in Java Memento PatternMemento Design Pattern in Java Visitor PatternVisitor Design Pattern in Java Strategy PatternStrategy Design Pattern in Java Template Method PatternTemplate Method Design Pattern in Java
Structural Patterns: Adapter PatternAdapter design pattern in Java Composite PatternComposite Design Pattern in Java Facade PatternFacade Design Pattern in Java Proxy Pattern Java Proxy Design Pattern in Java
Analysis of Patterns: Strategy vs State PatternStrategy Design Pattern versus State Design Pattern